A collection of identically typed objects kept in consecutive memory locations is called an array.
It can be used to store a collection of primitive data types like int, char, float, and so on, as well as derived and user-defined data types like pointers, structures, and so on.
Types of array in C++
- One dimensional array
- Multi dimensional array
One dimensional array
One-dimensional arrays, commonly referred to as 1-D arrays in C++, are arrays with just one dimension.
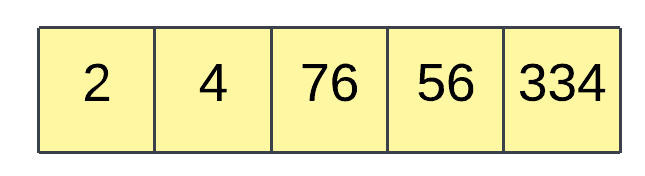
Multi dimensional array
In C++, arrays with more than one dimension are referred to as multi-dimensional arrays. Two and three-dimensional arrays are two common types of multidimensional arrays. Although we can declare arrays with more dimensions than 3D arrays, we should avoid doing so because they become very complex and take up a lot of space.
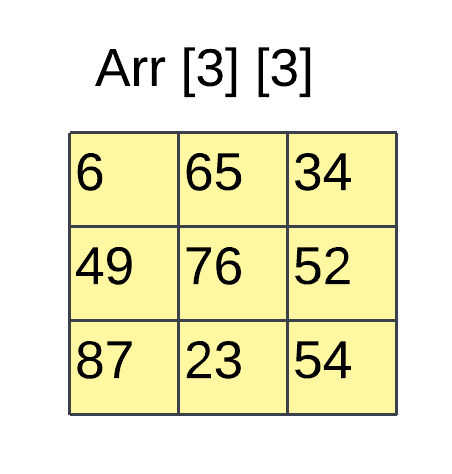
Array Declaration
syntax :-
Datatype array_name [ array_size ] ; // One Dimensional Array Or Datatype array_name [ array_size ] [ array_size2 ] ... ; // Multi dimensional Array
Array Initialization with Declaration
int arr[] = {10,20,30,40}
In this scenario we didn’t provided the number of elements ( Size of array ) but compiler will creates the array of size 4 . If we give the size in that case compiler will create array of that size.
Array Initialization after declaration
#include <iostream>
using namespace std;
int main() {
//Array Declaration
int arr[5];
/* Array Initialization after declaration using for loop */
for(int i=0;i<5;i++)
{
cin>>arr[i]; //2 4 76 56 334
}
/*Traverse :- Printing values Stored in the array */
for(int i=0;i<5;i++)
{
cout<<arr[i]<<" ";
}
return 0;
}
Output :- 2 4 76 56 334
Operations on Array
- Traverse
- Access
- Update
- Search
- Delete
- Insert
Accessing array elements
We can access array elements directly using the index of array element.
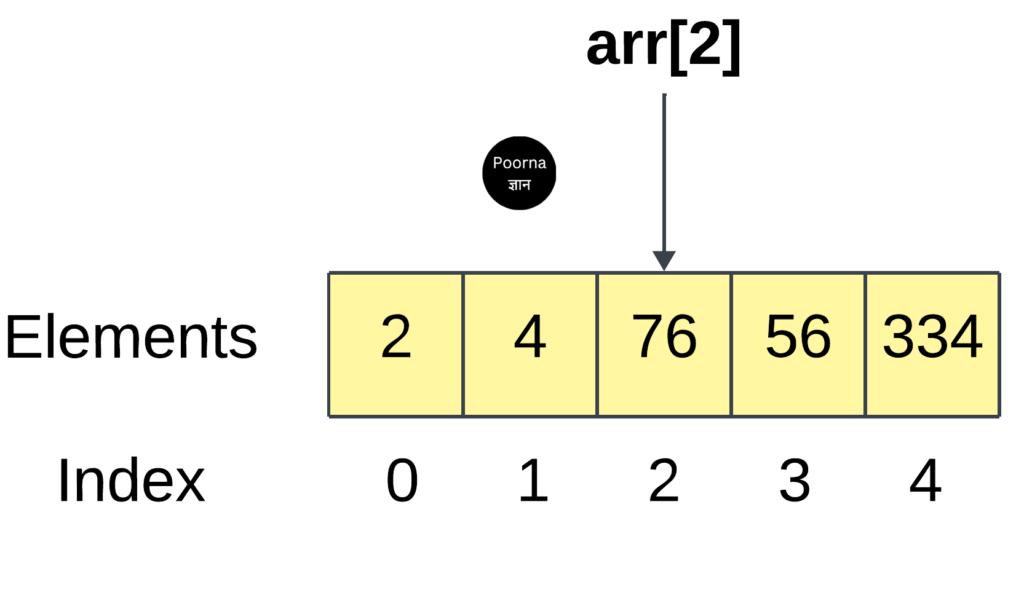
arr [2] ; // it will access element which is stored at index 2
array’s indexing always begins at 0, meaning that the initial element is located at index 0 and the final element is at N – 1, where N represents the total number of elements in the array.
Update array elements
In a manner similar to accessing an element, we can update the value of an element at the specified index
array_name [ index ] = new_value ;
Search element in array
Searching in a array can be done using 2 methods
- Linear Search
- Binary Search
In this Chapter we will learn Searching using linear search. In the linear search we traverse through the array and compare the array element with search element if we get element we will return true
#include<iostream>
using namespace std;
int main() {
//Array Declaration with initialization
int arr[5]={2,4,76,56,334};
int search=56;
int flag=0;
/*Traverse and compare the element with search term*/
for(int i=0;i<5;i++)
{
if(arr[i]==search)
{
cout<<search<<" is present in the array"<<endl;
flag=1;
break;
}
}
if(flag==0)
{
cout<<search<<" is not present in the array"<<endl;
}
return 0;
}
Output :- 56 is present in the array
Insert elements in array
- Insert at the middle of array
- Insert at the starting of the array
Insert at the middle of array
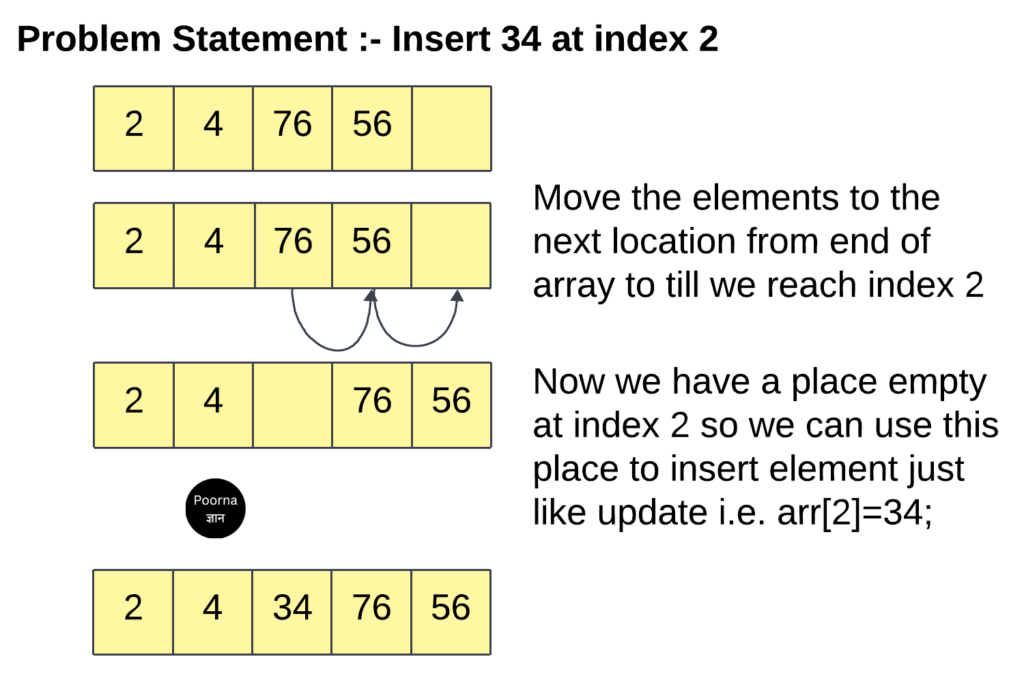
Insert at the beginning of array
Similar to insert at middle here we have to move elements to next location till we reach the index 0.
C++ Code for Insert at middle ( Kth Position ) and insert at beginning is given below :
#include<bits/stdc++.h>
using namespace std;
void print_array(int arr[],int n)
{
for(int i=0;i<n;i++)
{
cout<<i[arr]<<" ";//arr[i]
}
cout<<endl;
}
int insert_at_k_th_pos(int arr[],int n,int value,int k)
{
for(int i=n;i>=k;i--)
{
arr[i]=arr[i-1];
}
arr[k-1]=value;
n++;
return n;
}
int insert_at_start(int arr[],int n,int value)
{
for(int i=n;i>=1;i--)
{
arr[i]=arr[i-1];
}
arr[0]=value;
n++;
return n;
}
void create_array(int arr[],int n) // Taking Custom input from user to initialize array
{
cout<<" Enter All elements separated by space : ";
for(int i=0;i<n;i++)
{
cin>>arr[i];
}
}
int main()
{
int arr[1000],n,k,value; // Here we have declare max array size 1000
cout<<"enter number of elements below 1000 : ";
cin>>n;
create_array(arr,n);
cout<<" Printing Array : "<<endl;
print_array(arr,n);
cout<<"Enter a value which you want to insert at start : ";
cin>>value;
n=insert_at_start(arr,n,value);
print_array(arr,n);
cout<<"Please enter a position less than "<<n<<" where you want to insert value : ";
cin>>k;
cout<<"Enter a value which you want to insert at "<<k<<" th position : ";
cin>>value;
n=insert_at_k_th_pos(arr,n,value,k);
print_array(arr,n);
return 0;
}
Output :-
enter number of elements below 1000 : 5
Enter All elements separated by space : 2 4 56 76 34
Printing Array :
2 4 56 76 34
Enter a value which you want to insert at start : 1
1 2 4 56 76 34
Please enter a position less than 6 where you want to insert value : 3
Enter a value which you want to insert at 3 th position : 45
1 2 45 4 56 76 34
Part 1 :- Basics of Array
Part 2 :- Insertion in Array
Part 3 :- Deletion in Array
Part 4 :- Searching in array